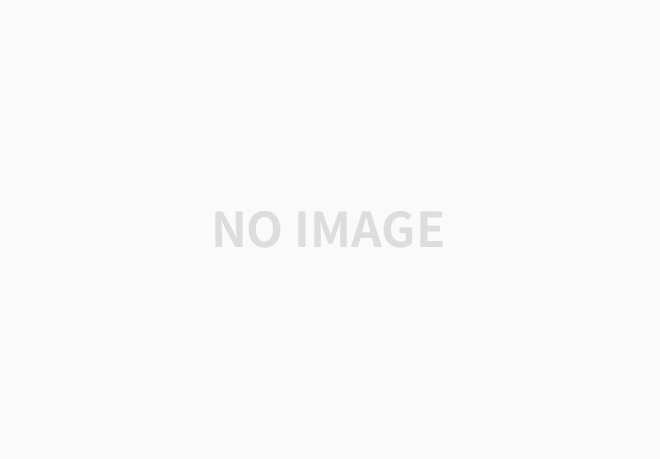
회귀분석이란?
- 회귀분석(Regression Analysis)은 특정 변수(독립변수)가 다른 변수(종속변수)에 어떠한 영향을 미치는지, 인과관계를 분석하는 것
- 변수 a 값이 변수 b 값에 영향을 주는 관계(인과관계)라면, 변수 a는 독립변수이고 변수 b는 종속변수이다.
- ex. 가격은 제품 만족도에 영향을 미치는가?
- 회귀분석 : 변수 간의 인과관계 분석 VS 상관관계분석 : 변수 간의 관련성 분석
- 회귀분석은 '통계분석의 꽃'이라고 불릴 만큼 가장 강력하고 많이 이용된다.
- 종속변수에 영향을 미치는 변수를 규명하고, 독립변수와 종속변수의 관련성 강도를 파악한다.
- '회귀 방정식'을 도출하여 회귀선을 추정 => 독립변수의 변화에 따른 종속변수 변화를 예측한다.
- 독립변수와 종속변수 모두 등간척도 또는 비율척도로 구성된다.
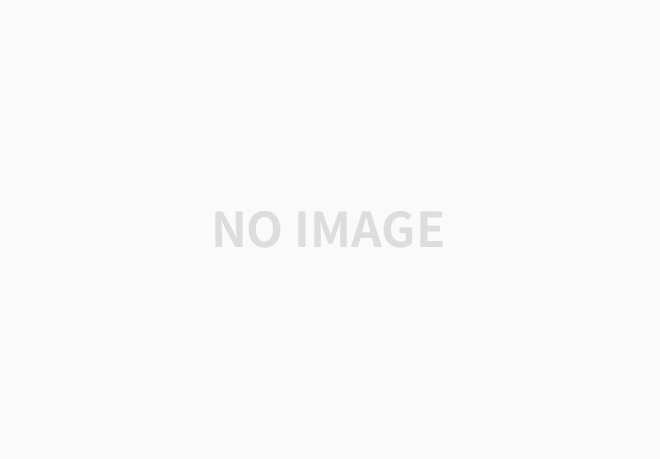
- 단순 회귀분석 : 독립변수와 종속변수가 각각 1개, 독립변수가 종속변수에 미치는 인과관계 분석
- 다중 회귀분석 : 여러 개의 독립변수가 1개의 종속변수에 미치는 영향 분석
- 분석 시 연구가설/귀무가설을 세워서 활용
선형 회귀방정식
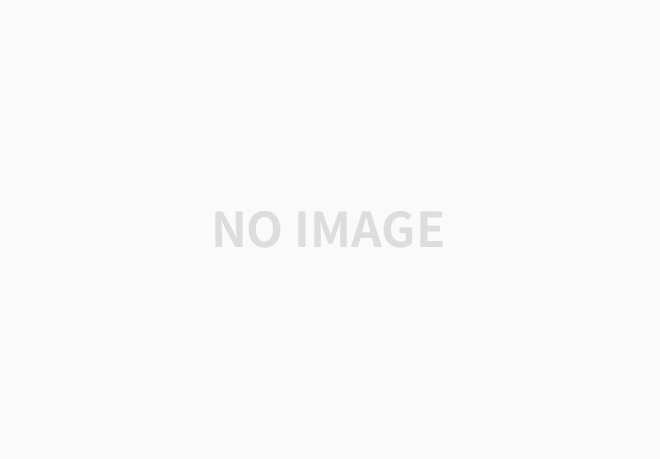
- 선형 회귀방정식(1차 함수) => 회귀선 추정
- Y = aX + b (Y : 종속변수, X : 독립변수, a : 기울기=회귀계수, b : 절편)
- 회귀방정식에 의해 종속변수 값 예측 (수치 예측)
- 최소자승법 적용 회귀선
- 회귀방정식에 의해서 그려진 y의 추세선
- 산포도 각 점의 위치를 기준으로 정중앙을 통과하는 회귀선 추정 방법
----------------------------------------- < 실습 > -----------------------------------------
"""
sklearn 회귀모델 생성
"""
import pandas as pd # csv file
from sklearn.linear_model import LinearRegression # 선형회귀모델 생성
from sklearn.model_selection import train_test_split # train/test set 생성
from sklearn.metrics import mean_squared_error # MSE : 평균제곱오차 - model 평가
# 1. dataset loading
iris = pd.read_csv("../data/iris.csv")
iris.info()
# 2. 변수(x, y) 선택 : 등간/비율 척도
cols = list(iris.columns)
cols
x_cols = cols[1:4]
y_col = cols[0]
x_cols # ['Sepal.Width', 'Petal.Length', 'Petal.Width']
y_col # 'Sepal.Length' - 임의로 고른 비율척도의 y변수
iris_df = iris[cols[:4]] # 분석에 불필요한 5번째 칼럼 제외한 데이터
# 3. train/test split (70 : 30)
iris_train, iris_test = train_test_split(iris_df, test_size = 0.3)
# train_test_split() 함수를 이용하면 train set과 test set 두개의 데이터가 test size로 나뉘어 랜덤으로 생성됨
iris_train.shape # (105, 4) - 학습용
iris_test.shape # (45, 4) - 검정용
# 4. 선형회귀모델 생성 : train
model = LinearRegression() # 생성자 -> 객체 생성
model.fit(X = iris_train[x_cols], y = iris_train[y_col]) # x=2~4번째 칼럼, y=1번째 칼럼
model.coef_ # 기울기 = [ 0.73784755, 0.79386389, -0.74633658]
model.intercept_ # 절편 = 1.4952262783130337
# 회귀방정식 = X * 기울기 + 절편
# 5. 모델 평가 : test
# 1) 모델 예측
y_pred = model.predict(X=iris_test[x_cols])
y_pred
len(y_pred) # 45
# 2) 정답
Y = iris_test[y_col]
Y
len(Y) # 45
# 3) MSE(평균제곱근오차) 평가
mse = mean_squared_error(y_true = Y, y_pred = y_pred)
print('mse =', mse)
# mse = 0.12271499851937004 => 이 값이 작을수록 모델의 예측력 높은 것
# 예측치 vs 정답
y_pred[:5] # [4.97725335, 6.09346843, 4.90346859, 5.63656341, 7.97599454]
Y[:5]
'''
29 4.7 <- 4.97725335 와 비교
54 6.5 <- 6.09346843 와 비교
30 4.8 <- 4.90346859 와 비교
32 5.2 <- 5.63656341 와 비교
117 7.7 <- 7.97599454 와 비교
'''
# 4) 상관계수
type(y_pred) # numpy.ndarray -> Series로 바꾸기
type(Y) # pandas.core.series.Series
y_pred = pd.Series(y_pred)
type(y_pred) # pandas.core.series.Series
# 이제 y_pred와 Y는 같은 Series 객체로 호환성 갖췄기 때문에 상관분석 가능
cor = Y.corr(y_pred)
cor
# 예측치와 관측치 이용해서 DF 생성
df = pd.DataFrame({'pred':y_pred, 'Y':Y})
df.info()
cor = df['pred'].corr(df['Y'])
cor # 0.0771843779171284 => 이 값이 클수록 모델의 예측력 높은 것
"""
제공되는 iris 데이터셋을 불러와서 회귀모델 만들고 평가하기
"""
from sklearn.linear_model import LinearRegression # 모델 생성
from sklearn.model_selection import train_test_split # train/test set
from sklearn.metrics import mean_squared_error # 모델 평가
from sklearn.datasets import load_iris # iris dataset
# 1. iris data load
iris = load_iris()
iris # 'data' : x(1~4), 'target' : y(5)
# 2. 변수 선택
iris_x = iris.data
iris_y = iris.target
iris_x.shape # (150, 4)
iris_y.shape # (150,)
# 3. train/test set(7 : 3)
x_train, x_test, y_train, y_test = train_test_split(iris_x, iris_y, test_size = 0.3)
# iris_x랑 iris_y 모두 7:3으로 나눠짐 => 실제 데이터 4개 만들어짐
x_train.shape # (105, 4)
x_test.shape # (45, 4)
y_train.shape # (105,)
y_test.shape # (45,)
# 4. 회귀모델 생성
model = LinearRegression()
model.fit(X = x_train, y = y_train)
# 5. 모델 예측치
y_pred = model.predict(X=x_test) # 예측치
y_true = y_test # 정답
# 6. 모델 평가 : mse, corr
mse = mean_squared_error(y_true, y_pred)
mse # 0.06450147901219713
import pandas as pd
df = pd.DataFrame({'y_pred' : y_pred, 'y_true' : y_true})
cor = df['y_pred'].corr(df['y_true'])
cor # 0.9613100872075921
----------------------------------------------- example -----------------------------------------------
'Python 과 머신러닝 > III. 머신러닝 모델' 카테고리의 다른 글
[Python 머신러닝] 7장. 앙상블 (Ensemble) - (2) RandomForest (0) | 2019.10.25 |
---|---|
[Python 머신러닝] 7장. 앙상블 (Ensemble) - (1) 앙상블의 개념 (0) | 2019.10.25 |
[Python 머신러닝] 6장. 분류 (Classification) (w/ scikit-learn) (1) | 2019.10.25 |
[Python 머신러닝] 5장. 회귀분석 - (2) 로지스틱 회귀분석 (0) | 2019.10.24 |
[Python 머신러닝] 지도학습과 비지도학습 (0) | 2019.10.23 |
댓글