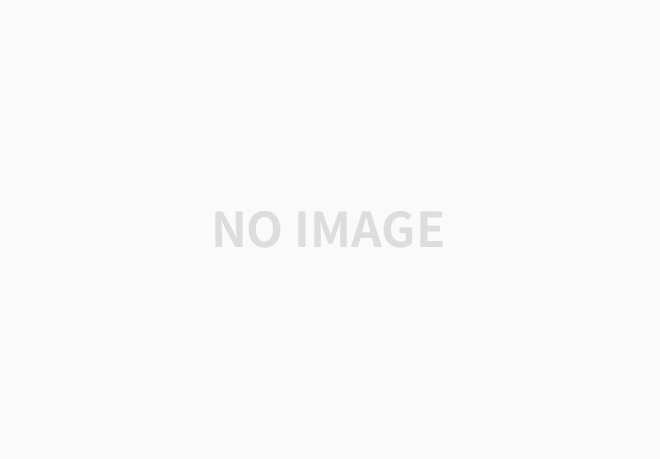
< 공부한 내용 >
1. Class 기초
2. self와 동적멤버변수
3. 기본 생성자
4. 클래스 상속
1. Class 기초
'''
함수 < 클래스 < 모듈(*.py) < 패키지
클래스(class)
- 함수의 모임
- 역할 : 여러 개의 함수와 공유 자료를 묶어서 객체 생성
- 구성 : 멤버 = 멤버변수(자료) + 메서드(함수), 생성자
- 유형 : 사용자 정의 클래스, 내장 클래스(python)
- 형식)
class 클래스이름 :
멤버변수 - 자료 저장
생성자 - 객체 생성
def 메서드( ) : - 자료 처리
'''
# < 함수와 클래스 비교 > - 함수정의 VS class 정의
# 1. 함수 정의
def calc(a, b) : # outer
# 자료(data)
x = a
y = b
# inner : plus
def plus() :
p = x + y
return p
# inner : minus
def minus() :
m = x - y
return m
return plus, minus
# 함수 호출
plus, minus = calc(100, 50)
print('plus =', plus()) # plus = 150
print('minus =', minus()) # minus = 50
# 2. class 정의
class Calc :
# 1. 멤버변수 (전역변수)
x = 0
y = 0
# 2. 생성자 : 객체 생성 + 멤버변수 초기화
def __init__(self, a, b): # a, b가 외부에서 받는 진짜 인수! 클래스 생성시 두 개의 인수 넣으면 됨
self.x = a
self.y = b
# 3. 메서드(함수) : 덧셈
def plus(self):
p = self.x + self.y
return p
# 3. 메서드(함수) : 뺄셈
def minus(self):
m = self.x - self.y
return m
# 객체 생성 : 생성자 이용
calc = Calc(100, 50) # calc 클래스 생성자
# 객체.멤버
print('plus=', calc.plus()) # plus= 150
print('minus=', calc.minus()) # minus= 50
# 객체2 생성 : 생성자 이용
calc2 = Calc(10, 5) # calc 클래스 생성자
# 객체2.멤버
print('plus=', calc2.plus()) # plus= 15
print('minus=', calc2.minus()) # minus= 5
# 객체의 주소 (다름)
print(id(calc), id(calc2)) # 66543056 66541904
# 3. 내장 클래스 : python에서 모듈 형태로 제공
import datetime # 방법1
from datetime import date # 방법2
help(date)
# Help on class date in module datetime:
# date(year, month, day) --> date object
today = datetime.date(2019, 10, 1) # 방법1 (모듈이름.함수이름)
today = date(2019, 10, 1) # 방법2
print(today) # 2019-10-01
# 객체.멤버변수
today.year
today.month
today.day
# 객체.메서드()
w = today.weekday() # 요일 반환
print('weekday=', w) # weekday= 1 (월요일=0 ~ 일요일=6)
2. self와 동적멤버변수
'''
1. self : 현재 클래스의 멤버를 호출하는 객체
* 멤버 = 멤버변수 + 메서드
self.멤버변수
self.메서드( )
2. 동적 멤버변수
- 필요한 경우 특정 메서드에서 생성하는 멤버변수
(미리 정의해두지 않고, 필요할 때, 그 때 만들어서 사용하는 변수)
- 형식) self.멤버변수 = 초기값
'''
class Car :
# 1. 멤버변수
name = None # null과 같은 뜻
door = cc = 0
# 2. 생성자
def __init__(self, name, door, cc):
self.name = name # 동적 멤버변수들
self.door = door
self.cc = cc
# 3. 메서드
def info(self):
if self.cc >= 3000 :
self.type = "대형" # 동적 멤버변수
else :
self.type = "중.소형" # 동적 멤버변수
self.display() # self.메서드() - 같은 클래스 안에 정의된 다른 메서드 사용
# 3. 메서드
def display(self):
print("%s는 %d cc이고(%s), 문짝은 %d개 이다."%(self.name, self.cc, self.type, self.door))
# 객체 생성 : 생성자 이용
car1 = Car("소나타", 4, 2000)
# 객체.멤버
car1.info() # 소나타는 2000 cc이고, 문짝은 4개 이다.
# 객체 생성
car2 = Car("그랜저", 4, 3500)
# 객체.멤버
car2.info() # 그랜저는 3500 cc이고(대형), 문짝은 4개 이다.
3. 기본 생성자
'''
기본 생성자
- 생성자를 생략하면 기본 생성자가 자동으로 만들어진다.
- 멤버변수 초기화가 없는 경우 생략 가능 -> 자동 생성
(생성자가 멤버변수를 초기화하는 역할을 하기 때문에 초기화 필요 없으면 생략)
- 모든 클래스는 반드시 생성자가 존재해야 한다.
'''
class default_cont :
# 멤버변수 없음
# 생성자 없음 : 기본 생성자
# 생략되어도 다음과 같은 모양의 생성자가 숨어있다고 생각하면 됨
'''
def __init__(self):
pass
'''
def data(self, x , y):
print('=== data method ===')
# 동적 멤버변수
self.x = x
self.y = y
def mul(self):
m = self.x * self.y
print('x * y = %d'%(m))
obj = default_cont() # 기본 생성자
obj.data(10,20) # === data method ===
obj.mul() # x * y = 200
# TV 클래스 정의
class TV :
# 1. 멤버변수 : 상태 (자료저장하는 역할)
channel = 10 # 채널
volume = 5 # 볼륨
power = False # off / on(True)
# 기본 생성자 : 생성자 생략
# 메서드 : 전원 관리(on/off)
def changePower(self):
self.power = not(self.power) # 부정
# 메서드 : 채널 up/down
def channelUp(self):
self.channel += 1 # 카운터
def channelDown(self):
self.channel -= 1
# 메서드 : 볼륨 up/down
def volumeUp(self):
self.volume += 1
def volumeDown(self):
self.volume -= 1
def display(self):
print(f"전원 상태 : {self.power}, 채널 번호 : {self.channel}, 볼륨 : {self.volume}")
# TV 객체 생성
tv1 = TV() # 기본 생성자(객체 생성만 가능)
tv1.display() # 전원 상태 : False, 채널 번호 : 10, 볼륨 : 5
tv1.changePower() # 전원 On
tv1.channelUp() # 채널 +1
tv1.volumeUp() # 볼륨 +1
tv1.display() # 전원 상태 : True, 채널 번호 : 11, 볼륨 : 6
'''
문) tv2 객체를 다음과 같이 생성하기
조건1> 전원 on
조건2> 채널 : 18번
조건3> 볼륨 : 9
조건4> tv 상태 출력하기
'''
tv2 = TV()
tv2.display()
tv2.changePower()
for i in range(8) :
tv2.channelUp()
tv2.volumeDown()
tv2.display()
'''
중첩함수 -> 클래스
outer(data) -> 멤버변수
inner(함수) -> 메서드
'''
# 계좌 클래스 정의
class Account :
# 정적 멤버변수
#balance = 0 # 잔액 => 이걸 메서드 안에서 사용할땐 nonlocal 붙이고 사용
# 생성자
def __init__(self, bal):
self.balance = bal # 동적 멤버변수 초기화
# 메서드 : 잔액 확인
def getBalance(self): # balance 확인 : getter() 역할
return self.balance
# 메서드 : 입금하기
def deposit(self, money): # balance += money : setter() 역할
self.balance += money # 잔액 수정
# 메서드 : 출금하기
def withdraw(self, money): # balance -= money : setter() 역할
if self.balance >= money :
self.balance -= money
else :
print("잔액이 부족합니다.")
# 계좌 객체 생성
acc = Account(1000) # 생성자
# 잔액 확인
print('잔액 :', acc.getBalance()) # 잔액 : 1000
# 입금하기
acc.deposit(15000)
# 잔액 확인
print('잔액 :', acc.getBalance()) # 잔액 : 16000
# 출금하기
acc.withdraw(6000)
# 잔액 확인
print('잔액 :', acc.getBalance()) # 잔액 : 10000
# 출금하기
acc.withdraw(20000) # 잔액이 부족합니다.
# 잔액 확인
print('잔액 :', acc.getBalance()) # 잔액 : 10000
4. 클래스 상속
'''
1. 클래스 상속(inheritance)
- 기존 클래스를 이용하여 새로운 클래스 생성 문법
- 부모클래스(old) -> 자식클래스(new)
- 상속 대상 : 멤버(멤버변수, 메서드)
- 생성자는 상속 대상이 아님
형식)
class new_class(old_class) :
pass
2. 메서드 재정의(method override)
- 상속관계에서만 나오는 용어
- 부모 원형 메서드를 자식에서 다시 작성하는 문법
'''
# 1. 클래스 상속(inheritance)
# 부모 클래스(old class)
class Sup :
# 1. 멤버변수
name = None
age = 0
# 2. 생성자
def __init__(self, name, age):
self.name = name
self.age = age
# 3. 메서드
def display(self):
print(f"name : {self.name}, age : {self.age}")
# 자식 클래스(new class)
class Sub(Sup) : # 자식클래스(부모클래스)
# 멤버변수, 메서드 상속됨 (생성자 제외 멤버들 상속)
# 1. 멤버변수
#name = None
#age = 0
# 2. 생성자
def __init__(self, name, age):
self.name = name
self.age = age
# 3. 메서드
#def display(self):
#print(f"name : {self.name}, age : {self.age}")
sub = Sub("자식클래스", "25")
print('name =', sub.name) # name = 자식클래스
print('age =', sub.age) # age = 25
sub.display() # name : 자식클래스, age : 25
# 2. 메서드 재정의(override) + 클래스 상속 응용
# 부모클래스(old)
class Parent :
# 멤버변수
name = job = None
# 생성자
def __init__(self, name, job):
self.name = name
self.job = job
# 메서드
def display(self):
print(f"name : {self.name}, job : {self.job}")
# 부모객체
p = Parent("부모", "공무원")
p.display() # name : 부모, job : 공무원
# 자식 클래스1
# 멤버변수, 메서드 상속됨(생성자 제외 멤버들 상속)
class Child1(Parent) :
age = 0 # 자식에서 새로 추가한 자식 멤버 변수
def __init__(self, name, job, age):
self.name = name
self.job = job
self.age = age # 자식 추가 멤버
# 메서드 재정의 (2개 -> 3개 확장 : '나이 출력 추가')
def display(self):
print(f"name : {self.name}, job : {self.job}, age : {self.age}")
ch1 = Child1("자식1", "군인", 25)
ch1.display() # name : 자식1, job : 군인, age : 25
# 자식 클래스2
# 멤버변수, 메서드 상속됨(생성자 제외 멤버들 상속)
class Child2(Parent) :
def __init__(self, name, job):
self.name = name
self.job = job
ch2 = Child2("자식2", "간호사")
ch2.display() # name : 자식2, job : 간호사
--------------------------------------------- example ---------------------------------------------
'Python 과 머신러닝 > I. 기초 문법' 카테고리의 다른 글
Python 기초 8장. DB 연동 (1) (0) | 2019.10.14 |
---|---|
Python 기초 7장. 파일 입출력 (0) | 2019.10.10 |
Python 기초 5장. 함수 (2) (0) | 2019.09.30 |
Python 기초 5장. 함수 (1) (0) | 2019.09.30 |
Python 기초 4장. 정규표현식 (0) | 2019.09.30 |
댓글